Object Constructors vs. Object Literals – Theory and Practice
- March 23, 2012
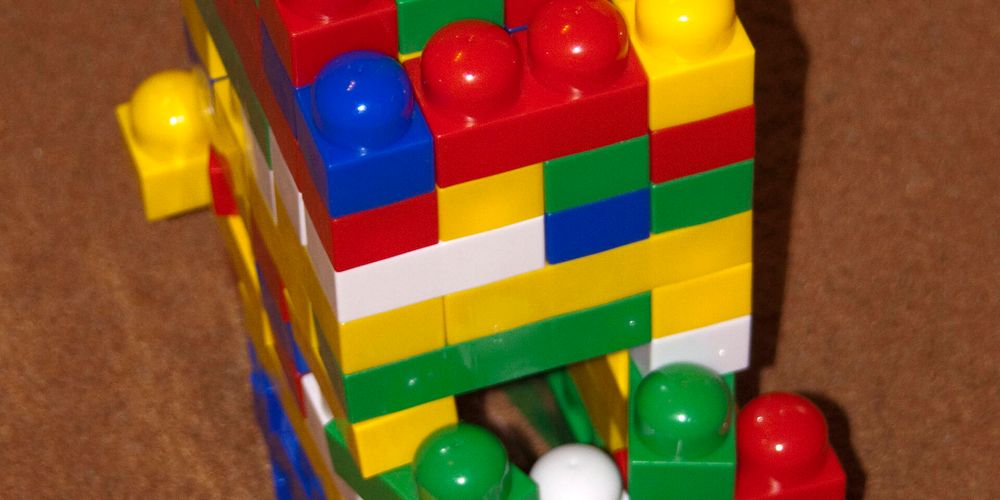
I have noticed recently some discussions about the comparison/contrast between object literals and objects created by a constructor. In the spirit of getting some information out there for people looking to better their skills in JavaScript and jQuery, I’ll go through some of the benefits of each method.
At its most basic, here is an object literal:
const literal = { key: 'value'};
And here is an object created with a constructor:
const constructed = new MyObject();
An object literal is an amalgamation of data and methods, immediately and publicly available once it has been created. It is a singleton, in the sense that because it is something you have manually created, it is the only instance of its type.
Objects created with a constructor have the benefit of inheriting the prototype of their class. Object literals do inherit the prototype of the default Object class, however they won’t inherit anything else you may have created that is custom to another object class.
Constructors also allow you to have private variables, something object literals do not. However, constructors are not immediately available, the way object literals are. They have to be instantiated (create an instance of).
Case in point:
function Person(name, gender, secret) {
this.name = name;
this.gender = gender;
this.legs = 2;
this.species = 'homo sapien';
const secret = secret;
this.blessYou = function() {
alert(this.name + ' says,"Bless you!");
};
}
const bob = new Person('Bob', 'male', 'stole $20');
const sally = {
name: 'Sally',
gender: 'female',
secret: 'watches Jersey Shore'
};
In the first case, with Bob, we have created an instance of a Person class that not only takes the information we provide to it (name, gender, and a secret), but it also defines a lot more for us. Species, the number of legs, and we have a function defined to give us a behaviour if someone sneezes (Maybe this is the event handler if a ‘sneeze’ event is dispatched).
In the second case, Sally only has the data that we know was being passed to the Person class, name, gender, and her secret. Also, we had to manually define each of those fields. However, for all we know, Sally has four legs and is an alpaca, who is also not as polite as Bob will be if someone sneezes. Also, Bob’s secret is hidden from us. We cannot call ‘bob.secret’ and find out about his thieving ways. However Sally’s secret (her Jersey Shore addiction) is publicly available.
Now, with the object constructor above, we could make a Tom, a Dick, a Jane, and three Mikey’s, and all would have the same behaviour as Bob. Sally, our rude four-legged alpaca however, stands alone. She doesn’t get these extra attributes and behaviours, as she was made with an object literal.
Now that I’ve given a really long-winded example of the difference between the two in theory let’s talk about it more in practice. Why would I prefer to use literals over constructors? Or why prefer constructors over literals?
The fact they are immediately available is the biggest thing. I will use object literals to pass data to an AJAX request, to build a cache for selecting DOM elements on a page, many dynamic things such as that. Business Logic elements could and should get their own constructors, as it may require multiple instances of it in order to accomplish my needs.
Which is one other point that is different between constructors and literals. To make a new object using a constructor, I have to create a new instance of it. With a literal, I can just copy it to a new variable. That doesn’t break the singleton mentality, we are creating a new singleton. The benefits of this are entirely implementation-dependent.
In summary, if you find yourself needing a quick one-off object for something (again, an example is sending post data to an AJAX request) then an object literal is a fantastic choice. They can be built programmatically as your business logic progresses, and can be copied to new variables quite easily. However, if you find yourself needing to extend a prototype for multiple instances of something, need to have public and private variables, then the constructor approach is definitely the best choice for you.
Are there other considerations? Feel free to chime in on the comments. I’m very open to lively discussion on this topic. I’ve just provided a rough overview here, with just some implementation considerations. I haven’t discussed memory footprints, or other factors.
Update: Here is an awesome article on Adobe’s website about the use of JS objects and singletons as a design pattern.